The GroupTaskbar is a panel level control that can be used for handling opened groups and items.
For example, like in the screenshot below, the GroupTaskbar object can be used to display the window objects launched in the taskbar mode of the MasterLayout.

The taskbar control supports events such as:
EventOnItemClose - when closing an item
EventOnItemSelect - when selecting an item
EventOnInitialize - on taskbar initialize
EventOnGroupClose - on taskbar group close
The events can be used to add custom ClientLogic to be able to implement custom business logic.
The GroupTaskbar control can be used togheter with the GlobalHooks in order to add and remove taskbar group and items when global events are fired, such as a window close event or a window launch event.
Some examples:
namespace akioma.osiv.taskbar {
/**
* Method executed on an taskbar item close
* @param Taskbar The Taskbar object
*/
export function TaskbarItemEventOnClose(Taskbar: akioma.swat.Taskbar) {
try {
const item = Taskbar.event.itemClosed as akioma.swat.TaskbarItem;
if (item.hasChanges) {
// check if we can migrate modal
window.akioma.message({
type: 'warning',
title: window.akioma.tran('messageBox.title.warning', { defaultValue: 'Warning' }),
text: window.akioma.tran('messageBox.text.unsavedChanges', { defaultValue: 'Closing the window will remove all the unsaved changes. Are you sure you want to continue?' }),
buttonText: window.akioma.tran('messageBox.button.yes', { defaultValue: 'close Window' }),
callback: function (result: boolean) {
if (result)
akioma.osiv.taskbar.closeAndSelectLastAvailable(item, Taskbar);
}
});
} else
akioma.osiv.taskbar.closeAndSelectLastAvailable(item, Taskbar);
} catch (e) {
console.error('Error on CloseTaskbarItem', e);
}
}
/**
* Method for closing the window and selecting the last active one
* @param {boolean} [item] The taskbar object for the window. If not specified, will be determined within the method
*/
export function closeAndSelectLastAvailable(item: akioma.swat.TaskbarItem, Taskbar: akioma.swat.Taskbar) {
const id = item.id;
const windowObject = akioma.swat.MasterLayout.findWindowById(id);
if (!item)
item = Taskbar.getItemById(id);
Taskbar.removeItem(windowObject.controller.opt.id);
windowObject.close();
const currentActive = Taskbar.getActiveItemByGroupId(item.parentid);
if (currentActive) {
Taskbar.selectItem(currentActive.id);
const activeWindow = akioma.swat.MasterLayout.findWindowById(currentActive.id);
if (activeWindow)
activeWindow.setActiveWindow();
}
}
}
* Event Listener for adding new taskbar items when a new container has been launched
* @param {ak_window} containerControl Window container launched
*/
akioma.swat.GlobalEmitter.on( akioma.swat.GlobalHooks.LAUNCH.AFTER_LOAD, ( containerControl : akioma.swat.Window | akioma.swat.Frame ) => {
const primaryDataSource = containerControl.dynObject.getLink( "PRIMARYSDO:TARGET" );
const isLoginScreen = window.app.sessionData.loginScreen === containerControl.controller.opt.name;
if ( containerControl.controller.bMainWindow || containerControl.controller.opt.modal || isLoginScreen )
return;
if ( akioma.ExternalScreen.externalScreenContainer === containerControl.controller.opt.name )
return;
if ( containerControl.controller.view !== "window" || !primaryDataSource )
return;
const primaryDataSourceControl = primaryDataSource.controller;
// after fill add taskbar item and group
primaryDataSourceControl.addAfterFillOnceCallback( () => {
const titleShortTemplateCompiled = primaryDataSourceControl.getRecordDescription( containerControl.controller.opt.titleShort );
const selfHdl = primaryDataSource.getValue( "selfhdl" );
buildone.addTaskbarItem( containerControl as akioma.swat.Window, selfHdl, titleShortTemplateCompiled );
} );
} );
/**
* Event listener called on an after save changes on a dataSource/businessEntity,
* used for updating the group title after a dataSource saveChanges operation
* @param {ak_businessEntity} dataSource Datasource object
*/
akioma.swat.GlobalEmitter.on( akioma.swat.GlobalHooks.DATASOURCE.AFTER_SAVE_CHANGES, async ( dataSource : akioma.swat.DataSource ) => {
const containerControl = dataSource.container?.controller;
const primaryDataSource = containerControl.dynObject?.getLink( "PRIMARYSDO:TARGET" );
if ( containerControl?.view !== "window" || !primaryDataSource )
return;
const TaskbarObject = akioma.swat.MasterLayout.getBaseLayoutObject().getFirstChildByType( "grouptaskbar" ) as akioma.swat.Taskbar;
const primaryDataSourceControl = primaryDataSource.controller;
const SelfHdl = primaryDataSource.getValue( "selfhdl" );
const res = await buildone.getRelatedPersonByUniqueKey( SelfHdl );
const resArray = res.split( "|" );
const parentId = resArray[ 0 ];
const title = resArray[ 1 ];
let groupData;
if (parentId) {
groupData = {
id: parentId,
title
};
} else {
const shortTemplate = containerControl.opt.titleShort || containerControl.opt.TITLE;
const titleShortTemplateCompiled = primaryDataSourceControl.getRecordDescription(shortTemplate);
groupData = {
id : TaskbarObject.getActiveGroupId(),
title : titleShortTemplateCompiled
};
}
TaskbarObject.updateGroup( groupData );
} );
/**
* Listener on desktop toggle mode to enable/disable header Taskbar
* @param {boolean} isDesktopMode Desktop state
*/
akioma.swat.GlobalEmitter.on( akioma.swat.GlobalHooks.DESKTOP.AFTER_TOGGLE, ( isDesktopMode : boolean ) => {
const taskbarObject = akioma.swat.MasterLayout.getBaseLayoutObject().getFirstChildByType( "grouptaskbar" ) as akioma.swat.Taskbar;
if ( !taskbarObject )
return;
if ( isDesktopMode )
taskbarObject.enable();
else
taskbarObject.disable();
} );
/**
* Event listener called after a window close event operation
* @param {ak_window} windowControl Window object closed
*/
akioma.swat.GlobalEmitter.on( akioma.swat.GlobalHooks.WINDOW.CLOSE, ( windowControl : akioma.swat.Window ) => {
const taskbarObject = akioma.swat.MasterLayout.getBaseLayoutObject().getFirstChildByType( "grouptaskbar" ) as akioma.swat.Taskbar;
taskbarObject.removeItem( windowControl.controller.opt.id );
} );
/**
* Event listener called on a focus event of a window, used for selecting the taskbar
* @param {ak_window} win Window object
*/
akioma.swat.GlobalEmitter.on( akioma.swat.GlobalHooks.WINDOW.FOCUS, ( windowControl : akioma.swat.Window ) => {
if ( !window.akioma.root || !window.akioma.root.dynObject )
return;
const taskbarObject = akioma.swat.MasterLayout.getBaseLayoutObject().getFirstChildByType( "grouptaskbar" ) as akioma.swat.Taskbar;
if ( !taskbarObject ) {
return;
}
taskbarObject.selectItem( windowControl.controller.opt.id, !windowControl.controller.disableFocusSelect );
} );
/**
* Event listener called on a window has errors state change, used for setting the taskbar has errors state
* @param windowControl
* @param hasErrors
*/
akioma.swat.GlobalEmitter.on( akioma.swat.GlobalHooks.WINDOW.HAS_ERRORS, ( { windowControl, hasErrors } : { windowControl : akioma.swat.Window, hasErrors : boolean } ) => {
const taskbarObject = akioma.swat.MasterLayout.getBaseLayoutObject().getFirstChildByType( "grouptaskbar" ) as akioma.swat.Taskbar;
if ( !taskbarObject )
return;
taskbarObject.setItemHasErrors( windowControl.controller.opt.id, hasErrors );
} );
/**
* Event listener called on a window has changes state change, used for setting the taskbar has changes state
* @param windowControl
* @param hasChanges
*/
akioma.swat.GlobalEmitter.on(akioma.swat.GlobalHooks.WINDOW.HAS_CHANGES, ( { windowControl, hasChanges } : { windowControl : akioma.swat.Window, hasChanges : boolean } ) => {
const baseLayoutObject = akioma.swat.MasterLayout.getBaseLayoutObject() as akioma.swat.Frame;
if ( !baseLayoutObject )
return;
const taskbarObject = baseLayoutObject.getFirstChildByType( "grouptaskbar" ) as akioma.swat.Taskbar;
if ( !taskbarObject )
return;
taskbarObject.setItemHasChanges( windowControl.controller.opt.id, hasChanges );
} );
External screens:

External screen items can be added from ClientLogic as seen in the example below:
This should be used together with launching the external screen using akioma.launchExternalScreen, see documentation of ExternalScreen
const taskbarObject = akioma.swat.MasterLayout.getBaseLayoutObject().getFirstChildByType( "grouptaskbar" ) as akioma.swat.Taskbar;
const activeGroupId : string = taskbarObject.getActiveGroupId();
const activeGroup = taskbarObject.getGroupById( activeGroupId );
taskbarObject.addExternalItem( {
// must be item id so the taskbar is recognized as external and displayed red
id : activeGroup.item_active,
title : response.MessageDetail
});
Styling for GroupTaskbar
The following SASS variables are available for customizing the appearance of the GroupTaskbar:
$taskbar_item_bgColor: #e4e4e4 !default;
$taskbar_item_active_bgColor: #394c61 !default;
$taskbar_item_external_screen_bgColor: #7a1d17 !default;
$taskbar_item_external_screen_color: #fff !default;
$taskbar_item_color: #000 !default;
$taskbar_item_active_color: #fff !default;
$taskbar_item_padding: 11px 40px 11px 10px !default;
$taskbar_item_max_width: 250px !default;
$taskbar_group_bgColor: #e4e4e4 !default;
$taskbar_group_active_bgColor: #394c61 !default;
$taskbar_group_color: #000 !default;
$taskbar_group_active_color: #fff !default;
$taskbar_group_width: 250px !default;
$taskbar_group_max_width: 250px !default;
$taskbar_group_border_bottom: solid 1px white !default;
$taskbar_group_content_min_width: 220px !default;
$taskbar_group_content_max_width: 220px !default;
$taskbar_group_content_padding: 10px 40px 10px 7px !default;
$taskbar_bgColor: #fff !default;
$taskbar_font_family: roboto, arial, helvetica !default;
$taskbar_close_icon_fill: #fff !default;
Taskbar (DataView template)
The Taskbar is the top section of the screen where all opened container controls are displayed as icons with a label. The purpose is the serve multi-tasking operations and the user can switch between these by a simple mouse-click.
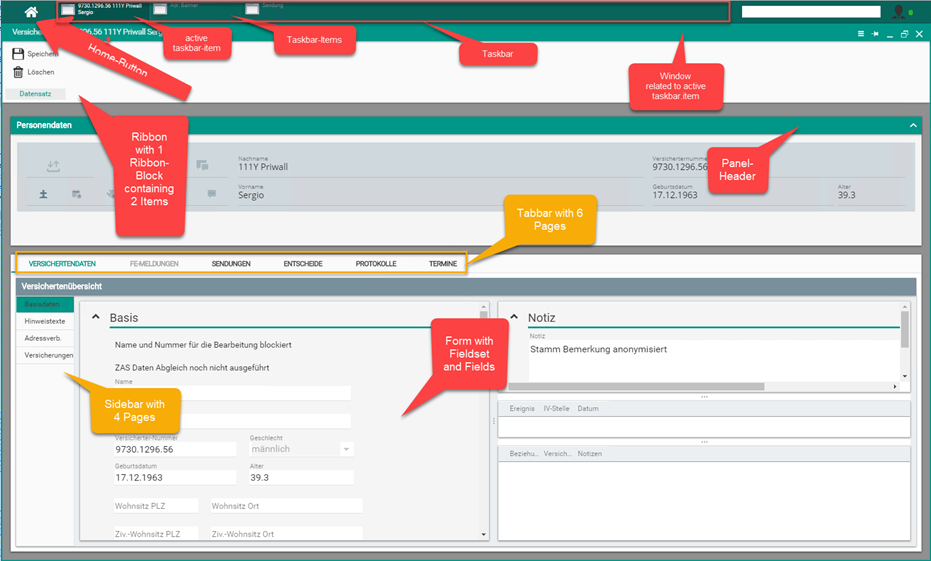